Basic file setup
Create a new Flutter project or open an existing one. We will add examples of each button in the main.dart file.
import 'package:flutter/material.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Flutter Input and Form Widgets")),
body: InputFormDemo(),
),
);
}
}
class InputFormDemo extends StatefulWidget {
@override
_InputFormDemoState createState() => _InputFormDemoState();
}
class _InputFormDemoState extends State<InputFormDemo> {
final _formKey = GlobalKey<FormState>(); // Form key for validation
String? _radioValue;
bool _checkboxValue = false;
bool _switchValue = false;
double _sliderValue = 20;
DateTime? _selectedDate;
TimeOfDay? _selectedTime;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(16.0),
child: Form(
key: _formKey,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [//PUT WIDGETS HERE
],
),
),
);
}
}
TextField
The TextField
widget is a basic input field for text
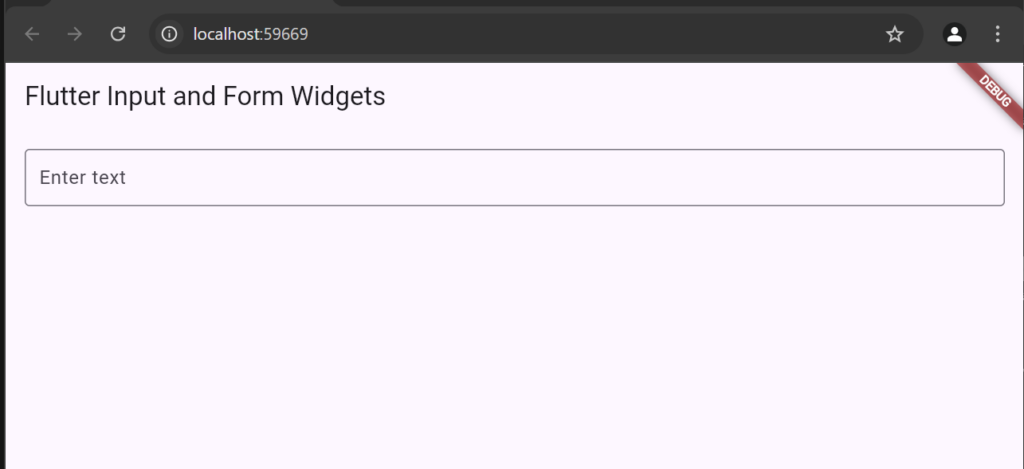
TextFieldWidget.dart
import 'package:flutter/material.dart';
class TextFieldWidget extends StatelessWidget {
final TextEditingController _controller = TextEditingController();
@override
Widget build(BuildContext context) {
return TextField(
controller: _controller,
decoration: InputDecoration(
labelText: "Enter text",
border: OutlineInputBorder(),
),
);
}
}
main.dart file
import 'package:flutter/material.dart';
import 'package:basic_widgets/TextFieldWidget.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Flutter Input and Form Widgets")),
body: InputFormDemo(),
),
);
}
}
class InputFormDemo extends StatefulWidget {
@override
_InputFormDemoState createState() => _InputFormDemoState();
}
class _InputFormDemoState extends State<InputFormDemo> {
final _formKey = GlobalKey<FormState>(); // Form key for validation
String? _radioValue;
bool _checkboxValue = false;
bool _switchValue = false;
double _sliderValue = 20;
DateTime? _selectedDate;
TimeOfDay? _selectedTime;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(16.0),
child: Form(
key: _formKey,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [TextFieldWidget()
],
),
),
);
}
}
CheckBoxWidget
The Checkbox
widget allows users to make a binary choice.
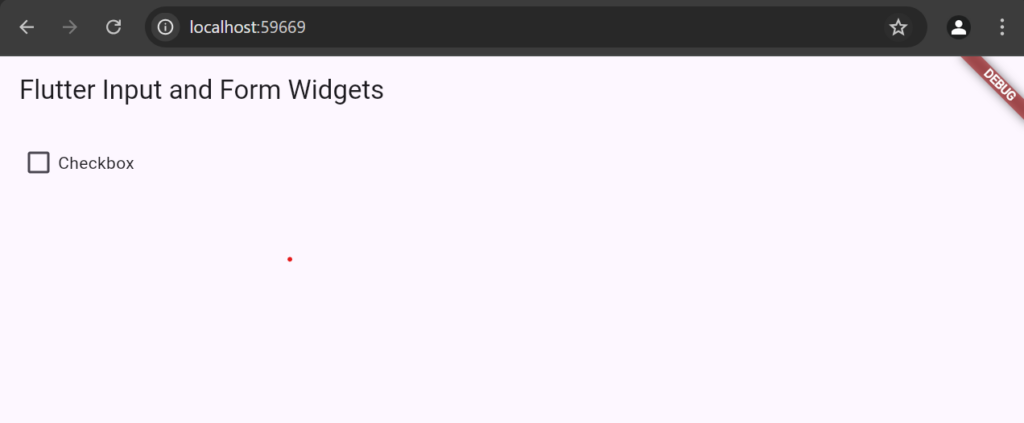
CheckboxWidget.dart file
import 'package:flutter/material.dart';
class CheckboxWidget extends StatelessWidget {
final bool value;
final ValueChanged<bool?> onChanged;
CheckboxWidget({required this.value, required this.onChanged});
@override
Widget build(BuildContext context) {
return Row(
mainAxisAlignment: MainAxisAlignment.start,
children: [
Checkbox(value: value, onChanged: onChanged),
Text("Checkbox"),
],
);
}
}
main.dart file
import 'package:flutter/material.dart';
import 'package:basic_widgets/CheckboxWidget.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Flutter Input and Form Widgets")),
body: InputFormDemo(),
),
);
}
}
class InputFormDemo extends StatefulWidget {
@override
_InputFormDemoState createState() => _InputFormDemoState();
}
class _InputFormDemoState extends State<InputFormDemo> {
final _formKey = GlobalKey<FormState>(); // Form key for validation
String? _radioValue;
bool _checkboxValue = false;
bool _switchValue = false;
double _sliderValue = 20;
DateTime? _selectedDate;
TimeOfDay? _selectedTime;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(16.0),
child: Form(
key: _formKey,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [CheckboxWidget(
value: _checkboxValue,
onChanged: (bool? value) {
setState(() {
_checkboxValue = value ?? false;
});
},
),
],
),
),
);
}
}
SwitchWidget
The Switch
widget is similar to the checkbox but with a slider-like toggle.
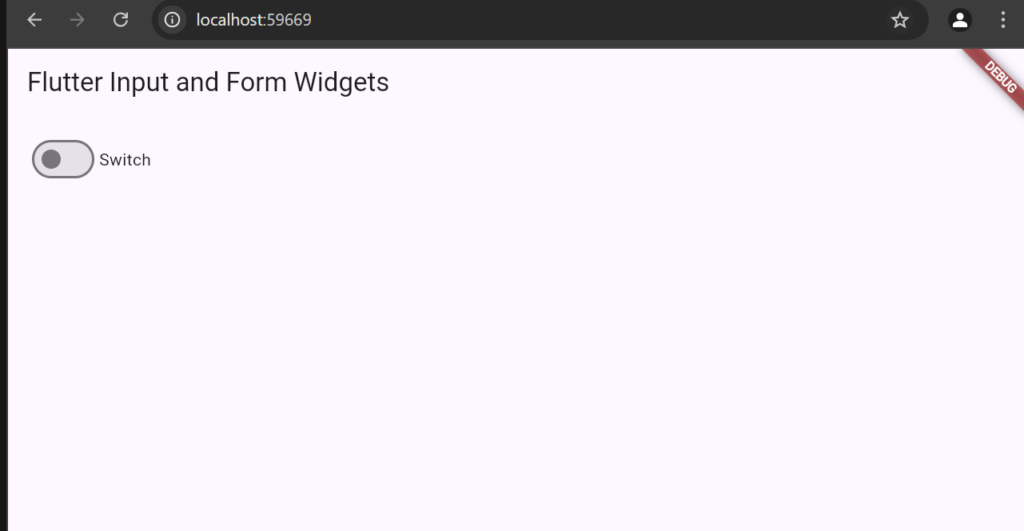
SwitchWidget.dart file
import 'package:flutter/material.dart';
class SwitchWidget extends StatelessWidget {
final bool value;
final ValueChanged<bool> onChanged;
SwitchWidget({required this.value, required this.onChanged});
@override
Widget build(BuildContext context) {
return Row(
mainAxisAlignment: MainAxisAlignment.start,
children: [
Switch(value: value, onChanged: onChanged),
Text("Switch"),
],
);
}
}
main.dart file
import 'package:flutter/material.dart';
import 'package:basic_widgets/SwitchWidget.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Flutter Input and Form Widgets")),
body: InputFormDemo(),
),
);
}
}
class InputFormDemo extends StatefulWidget {
@override
_InputFormDemoState createState() => _InputFormDemoState();
}
class _InputFormDemoState extends State<InputFormDemo> {
final _formKey = GlobalKey<FormState>(); // Form key for validation
String? _radioValue;
bool _checkboxValue = false;
bool _switchValue = false;
double _sliderValue = 20;
DateTime? _selectedDate;
TimeOfDay? _selectedTime;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(16.0),
child: Form(
key: _formKey,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [ SwitchWidget(
value: _switchValue,
onChanged: (bool value) {
setState(() {
_switchValue = value;
});
},
),
],
),
),
);
}
}
RadioWidget
The Radio
widget lets users choose one option from multiple choices.
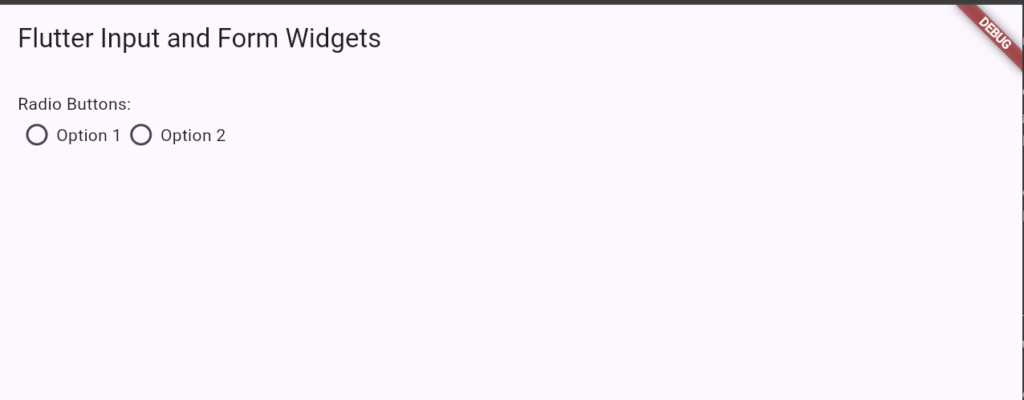
RadioButtonWidget.dart
import 'package:flutter/material.dart';
class RadioButtonWidget extends StatelessWidget {
final String? groupValue;
final ValueChanged<String?> onChanged;
RadioButtonWidget({required this.groupValue, required this.onChanged});
@override
Widget build(BuildContext context) {
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text("Radio Buttons:"),
Row(
children: [
Radio<String>(
value: "Option 1",
groupValue: groupValue,
onChanged: onChanged,
),
Text("Option 1"),
Radio<String>(
value: "Option 2",
groupValue: groupValue,
onChanged: onChanged,
),
Text("Option 2"),
],
),
],
);
}
}
main.dart file
import 'package:flutter/material.dart';
import 'package:basic_widgets/RadioButtonWidget.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Flutter Input and Form Widgets")),
body: InputFormDemo(),
),
);
}
}
class InputFormDemo extends StatefulWidget {
@override
_InputFormDemoState createState() => _InputFormDemoState();
}
class _InputFormDemoState extends State<InputFormDemo> {
final _formKey = GlobalKey<FormState>(); // Form key for validation
String? _radioValue;
bool _checkboxValue = false;
bool _switchValue = false;
double _sliderValue = 20;
DateTime? _selectedDate;
TimeOfDay? _selectedTime;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(16.0),
child: Form(
key: _formKey,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [ RadioButtonWidget(
groupValue: _radioValue,
onChanged: (String? value) {
setState(() {
_radioValue = value;
});
},
),
],
),
),
);
}
}
SliderWidget
The Slider
widget allows users to select a value from a range.
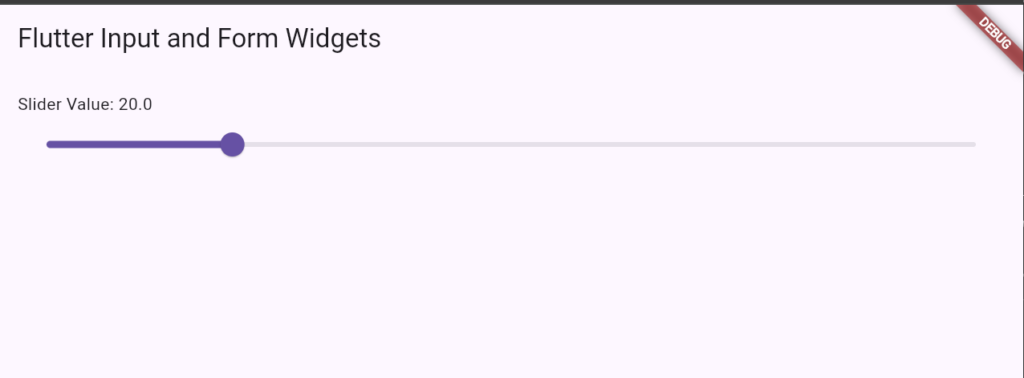
SliderWidget.dart
import 'package:flutter/material.dart';
class SliderWidget extends StatelessWidget {
final double value;
final ValueChanged<double> onChanged;
SliderWidget({required this.value, required this.onChanged});
@override
Widget build(BuildContext context) {
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text("Slider Value: ${value.toStringAsFixed(1)}"),
Slider(
value: value,
min: 0,
max: 100,
onChanged: onChanged,
),
],
);
}
}
main.dart file
import 'package:flutter/material.dart';
import 'package:basic_widgets/SliderWidget.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Flutter Input and Form Widgets")),
body: InputFormDemo(),
),
);
}
}
class InputFormDemo extends StatefulWidget {
@override
_InputFormDemoState createState() => _InputFormDemoState();
}
class _InputFormDemoState extends State<InputFormDemo> {
final _formKey = GlobalKey<FormState>(); // Form key for validation
String? _radioValue;
bool _checkboxValue = false;
bool _switchValue = false;
double _sliderValue = 20;
DateTime? _selectedDate;
TimeOfDay? _selectedTime;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(16.0),
child: Form(
key: _formKey,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [ SliderWidget(
value: _sliderValue,
onChanged: (double value) {
setState(() {
_sliderValue = value;
});
},
),
],
),
),
);
}
}
DatePickerWidget
The DatePicker
widget is invoked using the showDatePicker
function.
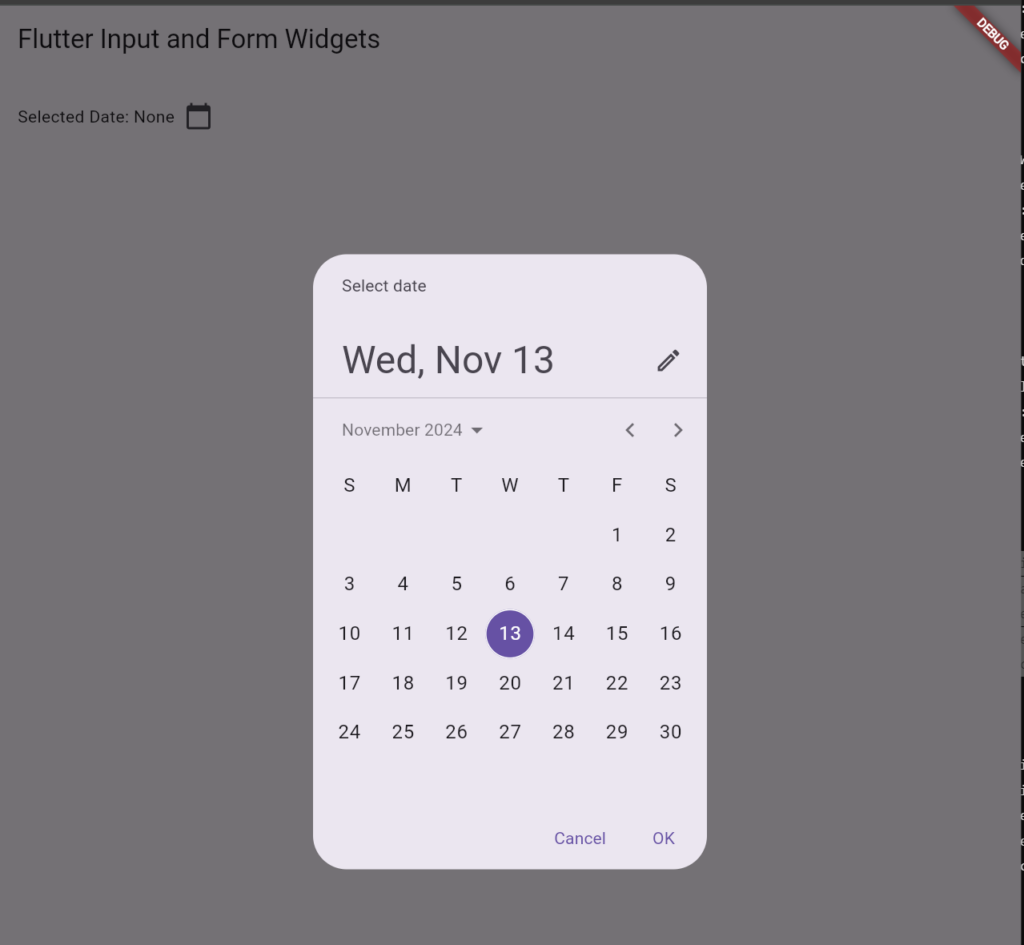
DatePickerWidget.dart
import 'package:flutter/material.dart';
class DatePickerWidget extends StatelessWidget {
final DateTime? selectedDate;
final ValueChanged<DateTime> onDateSelected;
DatePickerWidget({required this.selectedDate, required this.onDateSelected});
Future<void> _selectDate(BuildContext context) async {
final DateTime? picked = await showDatePicker(
context: context,
initialDate: selectedDate ?? DateTime.now(),
firstDate: DateTime(2000),
lastDate: DateTime(2100),
);
if (picked != null) {
onDateSelected(picked);
}
}
@override
Widget build(BuildContext context) {
return Row(
children: [
Text("Selected Date: ${selectedDate != null ? selectedDate!.toLocal().toString().split(' ')[0] : 'None'}"),
IconButton(
icon: Icon(Icons.calendar_today),
onPressed: () => _selectDate(context),
),
],
);
}
}
main.dart file
import 'package:flutter/material.dart';
import 'package:basic_widgets/DatePickerWidget.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Flutter Input and Form Widgets")),
body: InputFormDemo(),
),
);
}
}
class InputFormDemo extends StatefulWidget {
@override
_InputFormDemoState createState() => _InputFormDemoState();
}
class _InputFormDemoState extends State<InputFormDemo> {
final _formKey = GlobalKey<FormState>(); // Form key for validation
String? _radioValue;
bool _checkboxValue = false;
bool _switchValue = false;
double _sliderValue = 20;
DateTime? _selectedDate;
TimeOfDay? _selectedTime;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(16.0),
child: Form(
key: _formKey,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [ DatePickerWidget(
selectedDate: _selectedDate,
onDateSelected: (DateTime date) {
setState(() {
_selectedDate = date;
});
},
),
],
),
),
);
}
}
TimePickerWidget
The TimePicker
widget is invoked using the showTimePicker
function.
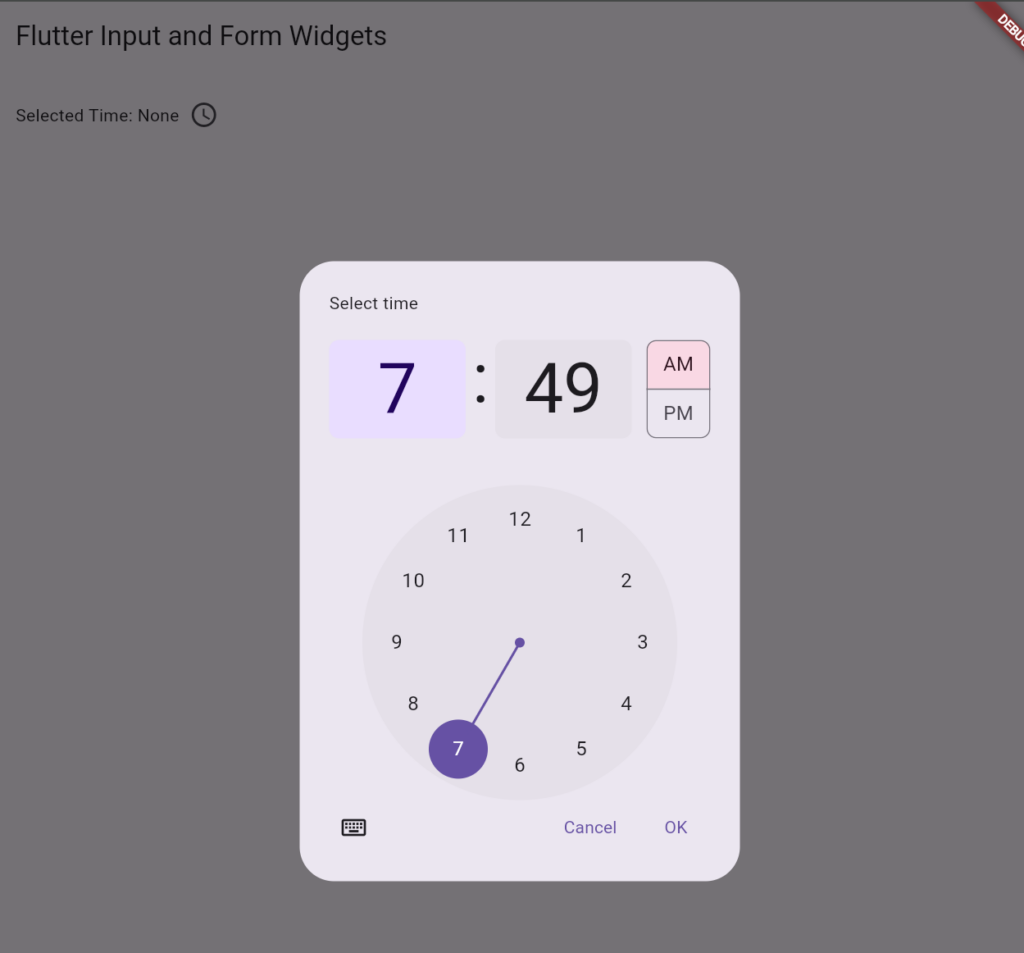
TimePickerWidget.dart file
import 'package:flutter/material.dart';
class TimePickerWidget extends StatelessWidget {
final TimeOfDay? selectedTime;
final ValueChanged<TimeOfDay> onTimeSelected;
TimePickerWidget({required this.selectedTime, required this.onTimeSelected});
Future<void> _selectTime(BuildContext context) async {
final TimeOfDay? picked = await showTimePicker(
context: context,
initialTime: selectedTime ?? TimeOfDay.now(),
);
if (picked != null) {
onTimeSelected(picked);
}
}
@override
Widget build(BuildContext context) {
return Row(
children: [
Text("Selected Time: ${selectedTime != null ? selectedTime!.format(context) : 'None'}"),
IconButton(
icon: Icon(Icons.access_time),
onPressed: () => _selectTime(context),
),
],
);
}
}
main.dart file
import 'package:flutter/material.dart';
import 'package:basic_widgets/TimePickerWidget.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Flutter Input and Form Widgets")),
body: InputFormDemo(),
),
);
}
}
class InputFormDemo extends StatefulWidget {
@override
_InputFormDemoState createState() => _InputFormDemoState();
}
class _InputFormDemoState extends State<InputFormDemo> {
final _formKey = GlobalKey<FormState>(); // Form key for validation
String? _radioValue;
bool _checkboxValue = false;
bool _switchValue = false;
double _sliderValue = 20;
DateTime? _selectedDate;
TimeOfDay? _selectedTime;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(16.0),
child: Form(
key: _formKey,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [ TimePickerWidget(
selectedTime: _selectedTime,
onTimeSelected: (TimeOfDay time) {
setState(() {
_selectedTime = time;
});
},
),
],
),
),
);
}
}
SubmitButtonWidget
Add a Submit
button to validate the form.
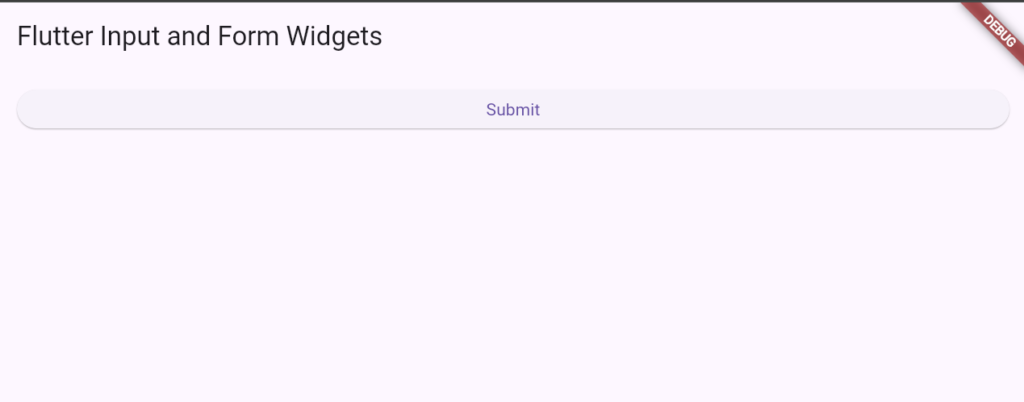
SubmitButtonWidget.dart file
import 'package:flutter/material.dart';
class SubmitButton extends StatelessWidget {
final GlobalKey<FormState> formKey;
SubmitButton({required this.formKey});
@override
Widget build(BuildContext context) {
return ElevatedButton(
onPressed: () {
if (formKey.currentState!.validate()) {
print("Form is valid");
} else {
print("Form is invalid");
}
},
child: Text("Submit"),
);
}
}
main.dart file
import 'package:flutter/material.dart';
import 'package:basic_widgets/SubmitButtonWidget.dart';
void main() {
runApp(MyApp());
}
class MyApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
home: Scaffold(
appBar: AppBar(title: Text("Flutter Input and Form Widgets")),
body: InputFormDemo(),
),
);
}
}
class InputFormDemo extends StatefulWidget {
@override
_InputFormDemoState createState() => _InputFormDemoState();
}
class _InputFormDemoState extends State<InputFormDemo> {
final _formKey = GlobalKey<FormState>(); // Form key for validation
String? _radioValue;
bool _checkboxValue = false;
bool _switchValue = false;
double _sliderValue = 20;
DateTime? _selectedDate;
TimeOfDay? _selectedTime;
@override
Widget build(BuildContext context) {
return Padding(
padding: const EdgeInsets.all(16.0),
child: Form(
key: _formKey,
child: Column(
crossAxisAlignment: CrossAxisAlignment.stretch,
children: [ SubmitButton(formKey: _formKey),
],
),
),
);
}
}