Overview
In this tutorial we will create a simple Flutter application that displays the output of simple Dart programs.
Final Output
Setting up the Flutter Project
Create a new Flutter Project
flutter create dart_basics
cd dart_basics
Folder Structure
lib/
├── main.dart
├── screens/
│ ├── variables_screen.dart
│ ├── functions_screen.dart
│ ├── classes_screen.dart
│ ├── collections_screen.dart
│ ├── control_flow_screen.dar
│ └── async_screen.dart
The main menu allows navigation to different tutorials.
main.dart
import 'package:flutter/material.dart';
import 'screens/variables_screen.dart';
import 'screens/functions_screen.dart';
import 'screens/classes_screen.dart';
import 'screens/collections_screen.dart';
import 'screens/async_screen.dart';
import 'screens/control_flow_screen.dart';
void main() {
runApp(DartBasicsApp());
}
class DartBasicsApp extends StatelessWidget {
@override
Widget build(BuildContext context) {
return MaterialApp(
debugShowCheckedModeBanner: false,
home: MainMenu(),
);
}
}
class MainMenu extends StatelessWidget {
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Dart Basics Tutorial')),
body: ListView(
children: [
ListTile(
title: Text('Variables and Constants'),
onTap: () {
Navigator.push(context, MaterialPageRoute(builder: (_) => VariablesScreen()));
},
),
ListTile(
title: Text('Functions'),
onTap: () {
Navigator.push(context, MaterialPageRoute(builder: (_) => FunctionsScreen()));
},
),
ListTile(
title: Text('Classes and Objects'),
onTap: () {
Navigator.push(context, MaterialPageRoute(builder: (_) => ClassesScreen()));
},
),
ListTile(
title: Text('Collections (Lists, Maps)'),
onTap: () {
Navigator.push(context, MaterialPageRoute(builder: (_) => CollectionsScreen()));
},
),
ListTile(
title: Text('Control Flow'),
onTap: () {
Navigator.push(context, MaterialPageRoute(builder: (_) => ControlFlowScreen()));
},
), // New menu option
ListTile(
title: Text('Asynchronous Programming'),
onTap: () {
Navigator.push(context, MaterialPageRoute(builder: (_) => AsyncScreen()));
},
),
],
),
);
}
}
Variables and Constants
This screen displays variable declarations, constant examples, and their outputs.
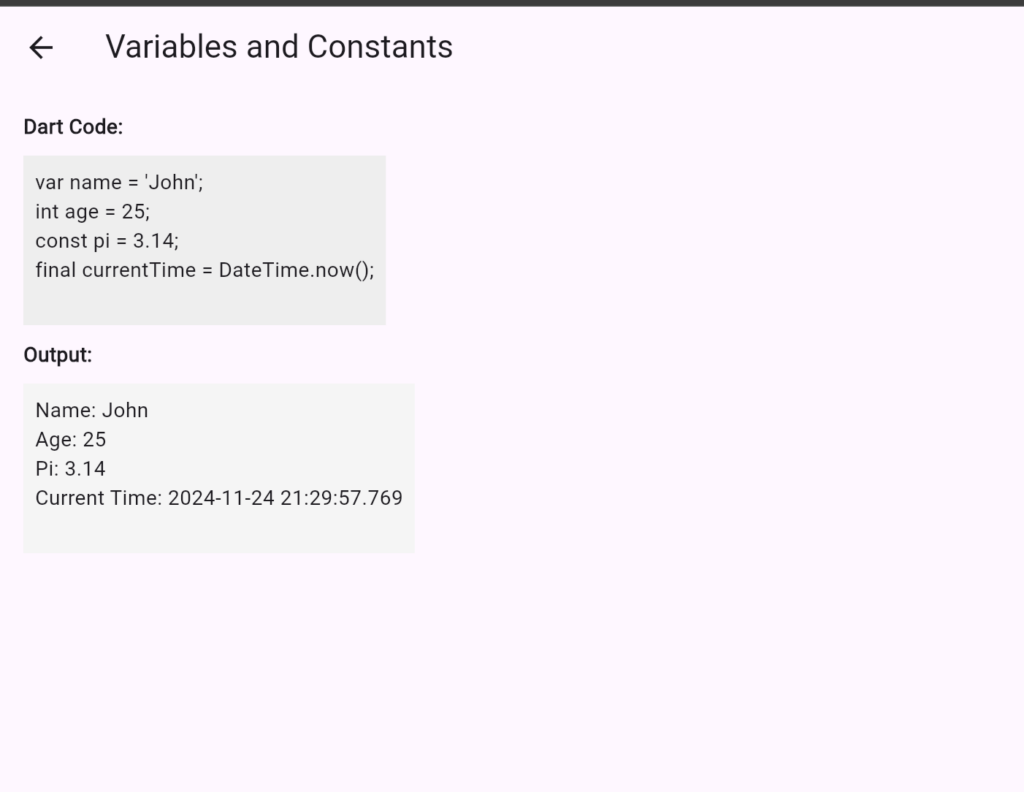
lib/screens/variables_screen.dart
import 'package:flutter/material.dart';
class VariablesScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
// Execute Dart logic
final name = 'John';
final age = 25;
const pi = 3.14;
final currentTime = DateTime.now().toString();
return Scaffold(
appBar: AppBar(title: Text('Variables and Constants')),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
'Dart Code:',
style: TextStyle(fontWeight: FontWeight.bold),
),
Container(
padding: EdgeInsets.all(8),
margin: EdgeInsets.symmetric(vertical: 10),
color: Colors.grey[200],
child: Text('''
var name = 'John';
int age = 25;
const pi = 3.14;
final currentTime = DateTime.now();
'''),
),
Text(
'Output:',
style: TextStyle(fontWeight: FontWeight.bold),
),
Container(
padding: EdgeInsets.all(8),
margin: EdgeInsets.symmetric(vertical: 10),
color: Colors.grey[100],
child: Text('''
Name: $name
Age: $age
Pi: $pi
Current Time: $currentTime
'''),
),
],
),
),
);
}
}
Functions
This screen demonstrates Dart functions, executes the logic, and shows the output.
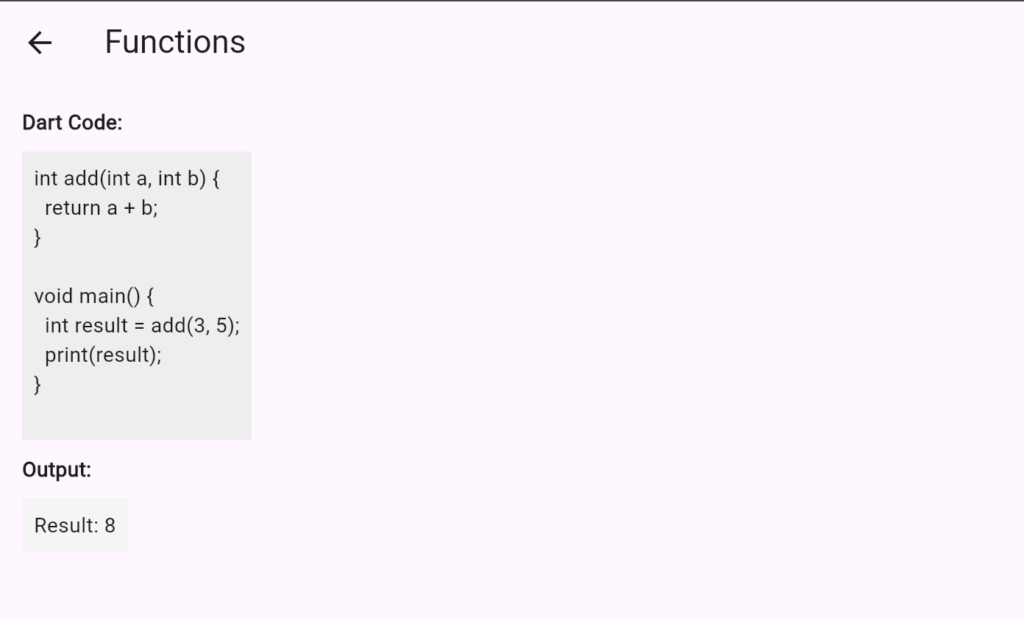
lib/screens/functions_screen.dart
import 'package:flutter/material.dart';
class FunctionsScreen extends StatelessWidget {
// Function to execute logic
int add(int a, int b) => a + b;
@override
Widget build(BuildContext context) {
final result = add(3, 5);
return Scaffold(
appBar: AppBar(title: Text('Functions')),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
'Dart Code:',
style: TextStyle(fontWeight: FontWeight.bold),
),
Container(
padding: EdgeInsets.all(8),
margin: EdgeInsets.symmetric(vertical: 10),
color: Colors.grey[200],
child: Text('''
int add(int a, int b) {
return a + b;
}
void main() {
int result = add(3, 5);
print(result);
}
'''),
),
Text(
'Output:',
style: TextStyle(fontWeight: FontWeight.bold),
),
Container(
padding: EdgeInsets.all(8),
margin: EdgeInsets.symmetric(vertical: 10),
color: Colors.grey[100],
child: Text('Result: $result'),
),
],
),
),
);
}
}
Classes and Objects
This screen shows how to define and use classes, executes the logic, and displays the output.
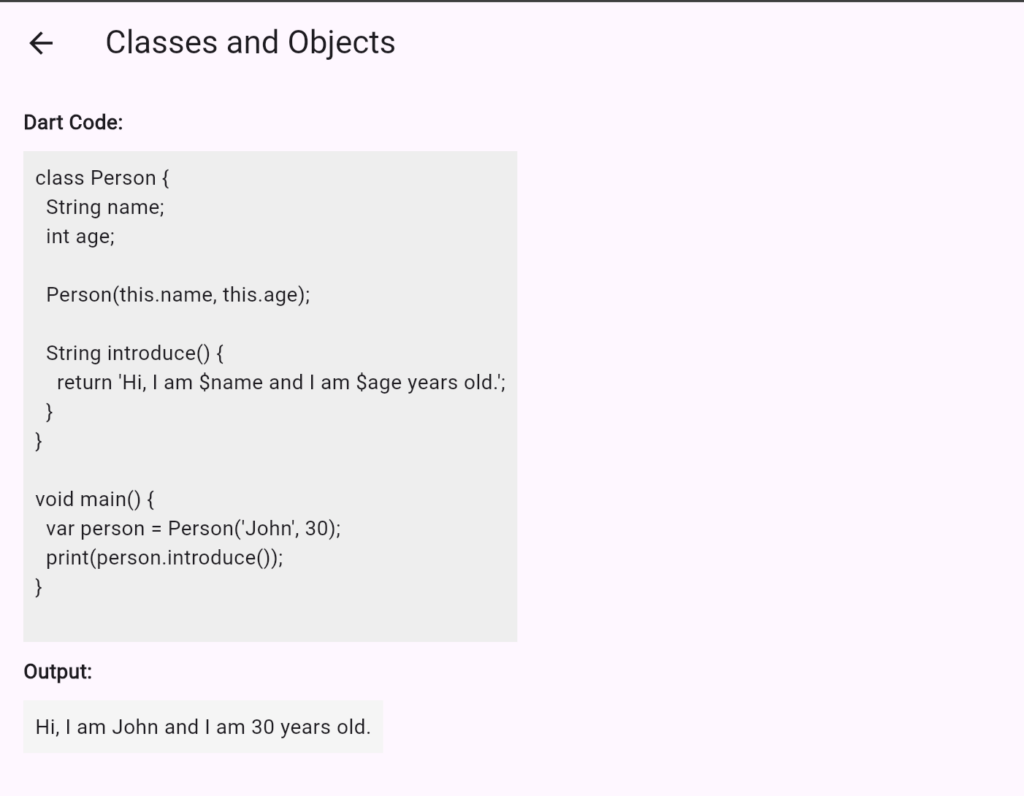
lib/screens/classes_screen.dart
import 'package:flutter/material.dart';
class Person {
final String name;
final int age;
Person(this.name, this.age);
String introduce() {
return 'Hi, I am $name and I am $age years old.';
}
}
class ClassesScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
final person = Person('John', 30);
return Scaffold(
appBar: AppBar(title: Text('Classes and Objects')),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
'Dart Code:',
style: TextStyle(fontWeight: FontWeight.bold),
),
Container(
padding: EdgeInsets.all(8),
margin: EdgeInsets.symmetric(vertical: 10),
color: Colors.grey[200],
child: Text('''
class Person {
String name;
int age;
Person(this.name, this.age);
String introduce() {
return 'Hi, I am \$name and I am \$age years old.';
}
}
void main() {
var person = Person('John', 30);
print(person.introduce());
}
'''),
),
Text(
'Output:',
style: TextStyle(fontWeight: FontWeight.bold),
),
Container(
padding: EdgeInsets.all(8),
margin: EdgeInsets.symmetric(vertical: 10),
color: Colors.grey[100],
child: Text(person.introduce()),
),
],
),
),
);
}
}
Collections
This screen demonstrates lists and maps, executes the logic, and displays the output.
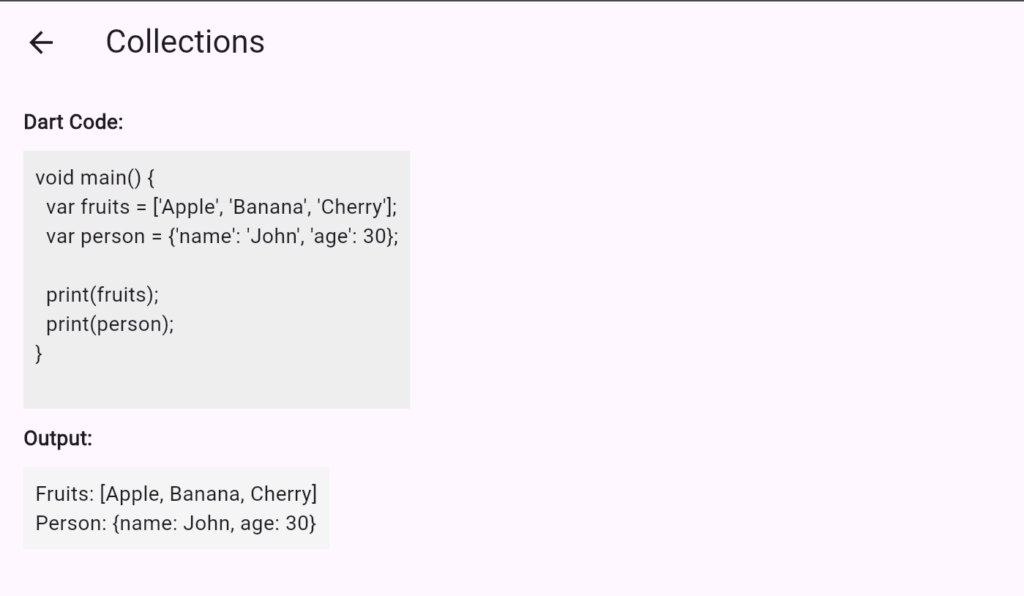
lib/screens/collections_screen.dart
import 'package:flutter/material.dart';
class CollectionsScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
// Dart logic
final fruits = ['Apple', 'Banana', 'Cherry'];
final person = {'name': 'John', 'age': 30};
return Scaffold(
appBar: AppBar(title: Text('Collections')),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
'Dart Code:',
style: TextStyle(fontWeight: FontWeight.bold),
),
Container(
padding: EdgeInsets.all(8),
margin: EdgeInsets.symmetric(vertical: 10),
color: Colors.grey[200],
child: Text('''
void main() {
var fruits = ['Apple', 'Banana', 'Cherry'];
var person = {'name': 'John', 'age': 30};
print(fruits);
print(person);
}
'''),
),
Text(
'Output:',
style: TextStyle(fontWeight: FontWeight.bold),
),
Container(
padding: EdgeInsets.all(8),
margin: EdgeInsets.symmetric(vertical: 10),
color: Colors.grey[100],
child: Text('Fruits: $fruits\nPerson: $person'),
),
],
),
),
);
}
}
Control Flow
This screen will include examples for if-else
, switch-case
, for
, while
, and do-while
loops.
lib/screens/control_flow_screen.dart
import 'package:flutter/material.dart';
class ControlFlowScreen extends StatelessWidget {
@override
Widget build(BuildContext context) {
// Example 1: If-Else
String checkAge(int age) {
if (age >= 18) {
return 'Adult';
} else {
return 'Minor';
}
}
// Example 2: Switch-Case
String dayOfWeek(int day) {
switch (day) {
case 1:
return 'Monday';
case 2:
return 'Tuesday';
case 3:
return 'Wednesday';
case 4:
return 'Thursday';
case 5:
return 'Friday';
case 6:
return 'Saturday';
case 7:
return 'Sunday';
default:
return 'Invalid day';
}
}
// Example 3: For Loop
List<int> forLoopExample() {
List<int> numbers = [];
for (int i = 1; i <= 5; i++) {
numbers.add(i);
}
return numbers;
}
// Example 4: While Loop
List<int> whileLoopExample() {
List<int> numbers = [];
int i = 1;
while (i <= 5) {
numbers.add(i);
i++;
}
return numbers;
}
// Example 5: Do-While Loop
List<int> doWhileLoopExample() {
List<int> numbers = [];
int i = 1;
do {
numbers.add(i);
i++;
} while (i <= 5);
return numbers;
}
return Scaffold(
appBar: AppBar(title: Text('Control Flow')),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: ListView(
children: [
buildExample(
'If-Else Example',
'''
void main() {
int age = 20;
if (age >= 18) {
print('Adult');
} else {
print('Minor');
}
}
''',
'Input: 20\nOutput: ${checkAge(20)}',
),
buildExample(
'Switch-Case Example',
'''
void main() {
int day = 5;
switch (day) {
case 1:
print('Monday');
break;
case 2:
print('Tuesday');
break;
// ...
}
}
''',
'Input: 5\nOutput: ${dayOfWeek(5)}',
),
buildExample(
'For Loop Example',
'''
void main() {
for (int i = 1; i <= 5; i++) {
print(i);
}
}
''',
'Output: ${forLoopExample()}',
),
buildExample(
'While Loop Example',
'''
void main() {
int i = 1;
while (i <= 5) {
print(i);
i++;
}
}
''',
'Output: ${whileLoopExample()}',
),
buildExample(
'Do-While Loop Example',
'''
void main() {
int i = 1;
do {
print(i);
i++;
} while (i <= 5);
}
''',
'Output: ${doWhileLoopExample()}',
),
],
),
),
);
}
Widget buildExample(String title, String code, String output) {
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
title,
style: TextStyle(fontSize: 16, fontWeight: FontWeight.bold),
),
SizedBox(height: 10),
Text('Dart Code:', style: TextStyle(fontWeight: FontWeight.bold)),
Container(
padding: EdgeInsets.all(8),
color: Colors.grey[200],
child: Text(code),
),
SizedBox(height: 10),
Text('Output:', style: TextStyle(fontWeight: FontWeight.bold)),
Container(
padding: EdgeInsets.all(8),
color: Colors.grey[100],
child: Text(output),
),
Divider(),
],
);
}
}
Explanation of Examples
- If-Else Example:
- Checks whether a person is an “Adult” or a “Minor” based on their age.
- Switch-Case Example:
- Maps a number (1–7) to a day of the week.
- For Loop Example:
- Iterates over numbers from 1 to 5 and displays them.
- While Loop Example:
- Iterates from 1 to 5 using a while loop.
- Do-While Loop Example:
- Similar to a while loop but guarantees the body executes at least once.
Asynchronous Programming
This screen demonstrates async functions, executes them, and displays the output.
lib/screens/async_screen.dart
import 'package:flutter/material.dart';
class AsyncScreen extends StatelessWidget {
Future<String> fetchData() async {
return Future.delayed(Duration(seconds: 1), () => 'Data fetched!');
}
@override
Widget build(BuildContext context) {
return Scaffold(
appBar: AppBar(title: Text('Asynchronous Programming')),
body: Padding(
padding: const EdgeInsets.all(16.0),
child: FutureBuilder<String>(
future: fetchData(),
builder: (context, snapshot) {
if (snapshot.connectionState == ConnectionState.waiting) {
return Center(child: CircularProgressIndicator());
}
return Column(
crossAxisAlignment: CrossAxisAlignment.start,
children: [
Text(
'Dart Code:',
style: TextStyle(fontWeight: FontWeight.bold),
),
Container(
padding: EdgeInsets.all(8),
margin: EdgeInsets.symmetric(vertical: 10),
color: Colors.grey[200],
child: Text('''
Future<String> fetchData() async {
return 'Data fetched!';
}
void main() async {
String data = await fetchData();
print(data);
}
'''),
),
Text(
'Output:',
style: TextStyle(fontWeight: FontWeight.bold),
),
Container(
padding: EdgeInsets.all(8),
margin: EdgeInsets.symmetric(vertical: 10),
color: Colors.grey[100],
child: Text(snapshot.data ?? ''),
),
],
);
},
),
),
);
}
}